Promises are used to handle asynchronous operations in JavaScript, providing an easier way to manage multiple asynchronous operations compared to using events and callback functions. Prior to promises, dealing with callbacks often resulted in callback hell, leading to unmanageable code. Callback hell occurs when multiple callback functions are nested within each other, making the code difficult to read and maintain. Promises offer a more structured approach to handling asynchronous operations, allowing for better code organization and error handling. By utilizing promises, developers can write cleaner and more maintainable code when dealing with asynchronous tasks in JavaScript.
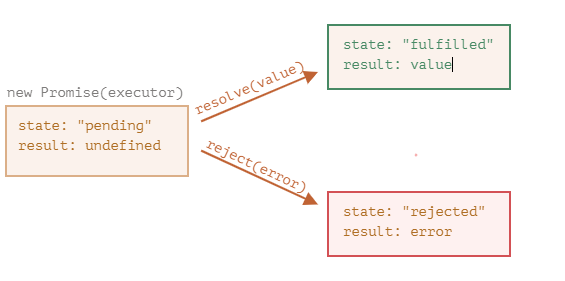
Parameters
- The promise constructor takes only one argument which is a callback function
- The callback function takes two arguments, resolve and reject
- Perform operations inside the callback function and if everything went well then call resolve.
- If desired operations do not go well then call reject.
Example
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Promise</h2>
<p id="demo"></p>
<script>
function myDisplayer(some) {
document.getElementById("demo").innerHTML = some;
}
let myPromise = new Promise(function(myResolve, myReject) {
let x = 0;
// some code (try to change x to 5)
if (x == 0) {
myResolve("OK");
} else {
myReject("Error");
}
});
myPromise.then(
function(value) {myDisplayer(value);},
function(error) {myDisplayer(error);}
);
</script>
</body>
</html>
Output:
