main.dart
import 'package:flutter/material.dart';
import 'package:flutter_application_1/myhomepages.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePages(),
);
}
}
myhomepages.dart
import 'package:flutter/material.dart';
import 'package:flutter/src/widgets/container.dart';
import 'package:flutter/src/widgets/framework.dart';
class MyHomePages extends StatefulWidget {
const MyHomePages({super.key});
@override
State<MyHomePages> createState() => _MyHomePagesState();
}
class _MyHomePagesState extends State<MyHomePages> {
final _formkey = GlobalKey<FormState>();
var name="";
var email="";
var password="";
final nameController = TextEditingController();
final emailController = TextEditingController();
final passwordController = TextEditingController();
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Flutter Container Example"),
),
body: Form(
key: _formkey,
child: Padding(
padding:const EdgeInsets.symmetric(vertical:40,horizontal:30)
,child: ListView(
children: [
TextFormField(
decoration:const InputDecoration(labelText:'Name:',
labelStyle: TextStyle(fontSize:40),
errorStyle: TextStyle(color:Colors.redAccent,fontSize:20 )),
controller: nameController,
validator: (value){
// print("validation me aarha hai");
if(value!.isEmpty){
// print("validation IF me aarha hai");
print(value);
return 'please Enter Name';
}
},
),
TextFormField(
decoration:const InputDecoration(labelText:'Email:',
labelStyle: TextStyle(fontSize:40)),
controller: emailController,
validator: (value){
if(value!.isEmpty){
return 'please Enter Email';
}
else if(!value.contains('@'))
{
return 'please Enter Valid Email';
}
},
),
TextFormField(
obscureText: true,
decoration:const InputDecoration(labelText:'password:',
labelStyle: TextStyle(fontSize:40)),
controller: passwordController,
validator: (value){
if(value!.isEmpty){
return 'please Enter password';
}
},
),
ElevatedButton(
onPressed: (){
if(_formkey.currentState!.validate()){
setState(() {
name=nameController.text;
email=emailController.text;
password=passwordController.text;
});
}
},
child:
Text('submit')),
Text('Name: $name'),
Text('Email: $email'),
Text('Password: $password'),
],
),
)
),
),
);
}
}
class _formkey {
static var currentState;
}
OutPut
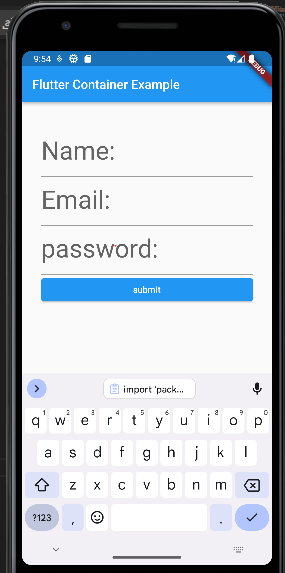