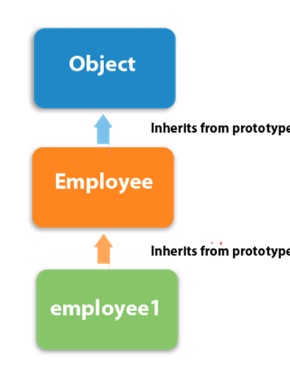
In JavaScript, the prototype object is a mechanism that allows you to add properties and methods to all instances of a particular object type. Every JavaScript object has a prototype property, which references the prototype object associated with it.
Here’s an example of using the prototype object to add properties and methods to objects:
// Constructor function for creating Person objects
function Person(name, age) {
this.name = name;
this.age = age;
}
// Adding a method to the prototype object of Person
Person.prototype.greet = function() {
console.log('Hello, my name is ' + this.name + ' and I am ' + this.age + ' years old.');
};
// Creating instances of Person
var person1 = new Person('John', 25);
var person2 = new Person('Jane', 30);
// Calling the greet method on the instances
person1.greet(); // Output: Hello, my name is John and I am 25 years old.
person2.greet(); // Output: Hello, my name is Jane and I am 30 years old.
In the example above, the Person
function acts as a constructor for creating Person
objects. We add properties name
and age
to each instance using the this
keyword inside the constructor.
Next, we use the prototype
property of the Person
function to add a greet
method to the prototype object. This means that all instances of Person
objects will have access to the greet
method through their prototype chain.
Note that the concept of prototypes is fundamental to JavaScript’s object-oriented programming model based on prototypal inheritance. It enables objects to inherit properties and methods from their prototypes, creating a chain of objects with shared functionality.
[…] https://www.devopsconsulting.in/blog/javascript-prototype-object/ […]