In Laravel, joining tables is a common task when working with relational databases. Laravel provides a fluent query builder that allows you to perform database operations, including joins, in an expressive way.
To join tables in Laravel, you can use the join
method on the query builder instance. The join
method accepts several arguments to define the join type and the tables involved in the join. Here’s an example of how you can perform a basic join in Laravel:
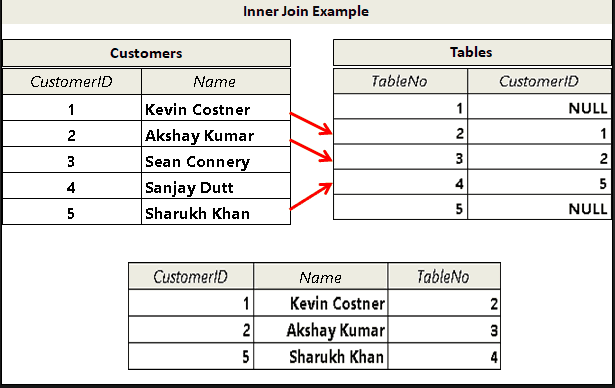
$users = DB::table('users')
->join('orders', 'users.id', '=', 'orders.user_id')
->select('users.*', 'orders.order_number')
->get();
In this example, we’re joining the “users” table with the “orders” table based on the “user_id” column. We’re selecting all columns from the “users” table and the “order_date” column from the “orders” table.
You can specify the join type by using methods such as join, leftJoin, rightJoin, or crossJoin, depending on your requirements.
leftJoin
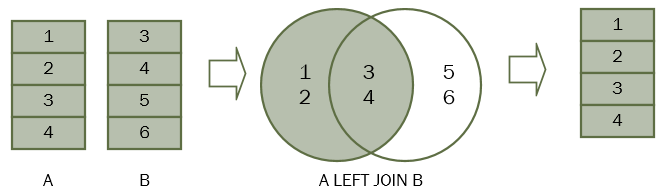
$users = DB::table('users')
->join('orders', 'users.id', '=', 'orders.user_id')
->join('payments', 'orders.id', '=', 'payments.order_id')
->select('users.name', 'orders.order_date', 'payments.amount')
->get();
In this case, we’re joining the “users” table with the “orders” table based on the “user_id” column and then joining the “payments” table with the “orders” table based on the “order_id” column. We’re selecting the user’s name, order date, and payment amount.