JavaScript Maps are a built-in data structure introduced in ECMAScript 6 (ES6) that allow you to store key-value pairs and retrieve the values based on their associated keys. Unlike regular JavaScript objects, Maps can have any value as a key, including objects, whereas object keys are converted to strings.
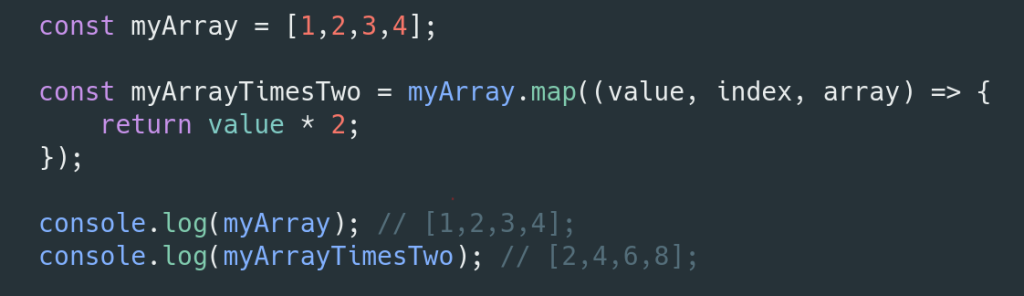
Example
// Creating a Map
const myMap = new Map();
// Adding key-value pairs to the Map
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
myMap.set('key3', 'value3');
// Retrieving values from the Map
console.log(myMap.get('key1')); // Output: "value1"
console.log(myMap.get('key2')); // Output: "value2"
// Checking if a key exists in the Map
console.log(myMap.has('key3')); // Output: true
// Getting the size of the Map
console.log(myMap.size); // Output: 3
// Deleting a key-value pair from the Map
myMap.delete('key2');
console.log(myMap.size); // Output: 2
// Iterating over the Map
myMap.forEach((value, key) => {
console.log(`${key} => ${value}`);
});
// Clearing the Map
myMap.clear();
console.log(myMap.size); // Output: 0
Maps provide several advantages over regular objects, such as maintaining the insertion order of the key-value pairs and providing methods to directly access the size, iterate over the entries, and easily delete all elements from the Map.
some real world example
// Creating a map to store inventory items and their quantities
const inventory = new Map();
// Adding inventory items
inventory.set('apple', 10);
inventory.set('banana', 15);
inventory.set('orange', 20);
inventory.set('grape', 5);
// Iterating over the inventory using forEach loop
inventory.forEach((quantity, item) => {
console.log(`Item: ${item}, Quantity: ${quantity}`);
});
// Output:
// Item: apple, Quantity: 10
// Item: banana, Quantity: 15
// Item: orange, Quantity: 20
// Item: grape, Quantity: 5
[…] JavaScript Maps […]
[…] JavaScript Maps […]