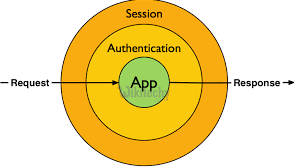
In Laravel, sessions provide a way to store data across multiple requests for a specific user. Laravel handles session management using the Illuminate\Session package.
Here’s a brief overview of how sessions work in Laravel:
- Starting a Session:
To start a session, Laravel automatically creates a session for each incoming request. You don’t need to manually start the session. - Storing Data in the Session:
You can store data in the session using the session helper or the Request instance. For example, to store a value in the session:
// Using the session helper
session(['key' => 'value']);
// Using the Request instance
$request->session()->put('key', 'value');
Retrieving Data from the Session:
You can retrieve data from the session using the session helper or the Request instance. For example:
What is Function of JPG to EPS Online tool
// Using the session helper
$value = session('key');
// Using the Request instance
$value = $request->session()->get('key');
Flash Data:
Flash data is a type of session data that is available only for the next request. You can flash data to the session using the session helper or the Request instance. For example:
// Using the session helper
session()->flash('key', 'value');
// Using the Request instance
$request->session()->flash('key', 'value');
Deleting Data from the Session:
To remove specific data from the session, you can use the forget method. For example:
session()->forget('key');
Destroying a Session:
If you want to completely destroy the session and remove all associated data, you can use the flush method. For example:
session()->flush();
These are some basic operations related to Laravel sessions. You can find more information in the official Laravel documentation: https://laravel.com/docs/session
[…] https://www.devopsconsulting.in/blog/laravel-sessions/ […]
[…] Laravel – sessions […]